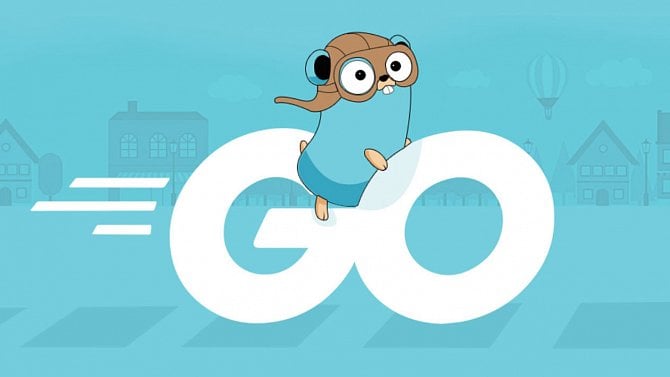
V najnovšej verzii programovacieho jazyka Go bol do štandardnej knižnice pridaný balíček iter, ktorý umožňuje vytvárať vlastné iterátory. V rámci týchto zmien boli pridané nové funkcie do balíčkov maps
a slices
. Balíček slices
má tieto nové funkcie:
- All
- Values
- Backward
- Collect
- AppendSeq
- Sorted
- SortedFunc
- SortedStableFunc
- Chunk
Nasledujúci príklad ukazuje použitie niektorých nových funkcií pre výrezy:
package main import ( "fmt" "slices" ) func main() { vals := []int{1, 2, 3, 4, 5, 6, 7, 8} for val := range slices.Backward(vals) { fmt.Println(val) } fmt.Println("---------------------------") for val := range slices.Chunk(vals, 2) { fmt.Println(val) } fmt.Println("---------------------------") words := []string{"sky", "ten", "water", "forest", "cup"} for i, v := range slices.All(words) { fmt.Println(i, ":", v) } fmt.Println("---------------------------") it := slices.Values(words) sorted_words := slices.Sorted(it) for _, word := range sorted_words { fmt.Println(word) } }
Balíček maps
má tieto nové funkcie:
- All
- Keys
- Values
- Insert
- Collect
Nasledujúci príklad ukazuje použitie niektorých nových funkcií pre mapy:
package main import ( "fmt" "maps" ) func main() { countries := map[string]string{ "sk": "Slovakia", "ru": "Russia", "de": "Germany", "no": "Norway", } for k := range maps.Keys(countries) { fmt.Println(k, ": ", countries[k]) } for v := range maps.Values(countries) { fmt.Println(v) } fmt.Println("------------------------") m1 := map[string]string{"po": "Poland"} m2 := map[string]string{"ro": "Romania"} it := maps.All(countries) maps.Insert(m1, it) maps.Insert(m2, it) countries2 := maps.Collect(it) for k, v := range maps.All(countries2) { fmt.Println(k, v) } }